Natas6-->Natas9
We continue on with the next 3 challenges for natas, focused on PHP!
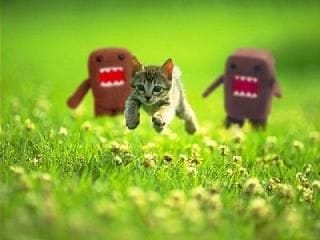
Review
We're continuing our series of the Natas challenges. The previous post covers the Natas4-->Natas6 challenges, which involved leveraging a local proxy to edit request headers. These next few challenges will focus on PHP, a popular server side scripting language.
Natas6-->Natas7
Website: http://natas6.natas.labs.overthewire.org/ ; Access Credentials: natas6:fOIvE0MDtPTgRhqmmvvAOt2EfXR6uQgR
Once authenticated, view the page and click on the "View sourcecode" hyperlink. There's some PHP code, the comments are my own, for a quick overview of the code:
<?
# imports another file, called secret.inc
include "includes/secret.inc";
# If a POST is made using the submit element
if(array_key_exists("submit", $_POST)) {
# If the secret submitted equals the hardcoded value $secret
# give the password!
if($secret == $_POST['secret']) {
print "Access granted. The password for natas7 is <censored>";
# Otherwise, give an response of wrong secret
} else {
print "Wrong secret";
}
}
?>
The value of $secret
is of interest to us, since it doesn't appear within the code, we should look for it elsewhere, mainly within includes/secret.inc file. Navigate to that link, which may appear blank, but when reviewing the source code of that page, shows the secret value of $secret = "FOEIUWGHFEEUHOFUOIU"
. Submit the string as the secret value at the main page of the natas6 site to retrieve the password: jmxSiH3SP6Sonf8dv66ng8v1cIEdjXWr
.
Natas7-->Natas8
Website: http://natas7.natas.labs.overthewire.org ; Access Credentials: natas7:jmxSiH3SP6Sonf8dv66ng8v1cIEdjXWr
Browsing to the site after authentication, open up the Developer Tools (CTRL+SHIFT+I
in Firefox). Within the Inspector tab, right click on the opening <body>
element, and select Expand All to review the source code. There's a hint within a comment of the source code (Line 21):
<!-- hint: password for webuser natas8 is in /etc/natas_webpass/natas8 -->
Look at the rest of the website source code. Note the two links, which use a page
parameter to reference two other pages, presumably local files, home
and about
. Click on one of the links, and it brings you to the main page. Note that the hyperlink in the browser has changed from the /
URI, to /index.php?page=about
. Change the value of about
to a bogus value, such as zentester
(more awesome, than bogus, but nonetheless) and an error appears:
Warning: include(zentester): failed to open stream: No such file or directory in /var/www/natas/natas7/index.php on line 21
Warning: include(): Failed opening 'zentester' for inclusion (include_path='.:/usr/share/php') in /var/www/natas/natas7/index.php on line 21
The error further confirms that it is reading local files on the system. A vulnerability type is local file inclusion. The vulnerability allows an attacker to read arbitrary files on the server running the website. It can be inferred based on both the source code command and the error message, and confirmed using tools such as Wappalyzer, that the server OS is Linux (Ubuntu specifically). set the value of the page
parameter to the natas8 file ( page=/etc/natas_webpass/natas8
), and retrieve the password: a6bZCNYwdKqN5cGP11ZdtPg0iImQQhAB
.
Natas8-->Natas9
Website: http://natas8.natas.labs.overthewire.org ; Access Credentials: natas8:a6bZCNYwdKqN5cGP11ZdtPg0iImQQhAB
The home page is similar to the Natas6 challenge, with some PHP code:
<?
$encodedSecret = "3d3d516343746d4d6d6c315669563362";
function encodeSecret($secret) {
return bin2hex(strrev(base64_encode($secret)));
}
if(array_key_exists("submit", $_POST)) {
if(encodeSecret($_POST['secret']) == $encodedSecret) {
print "Access granted. The password for natas9 is <censored>";
} else {
print "Wrong secret";
}
}
?>
However, instead of having a hard-coded secret on a different page, all of the code needed is within this block.
- The function takes the
$secret
value, base64 encodes, reverses the string and then converts the binary representation of the string to hexadecimal. - The if statement checks if the value of the
secret
variable provided in the POST request matches the$encodedSecret
, which is a hard-coded value.
This means to solve the answer, we need to create a reverse function.
PHP Reverse Function
In the spirit of using the same language that the challenge is written in, make your own PHP code, and use a PHP sandbox such as https://onlinephp.io/. See the "Alternative Solutions" section below on how to solve using the CLI.
<?php
$encodedSecret="3d3d516343746d4d6d6c315669563362";
function decodeSecret($encodedSecret) {
return base64_decode(strrev(hex2bin($encodedSecret)));
}
print decodeSecret($encodedSecret)
?>
The code creates a function, which does the reverse of the encodeSecret
function of the website. Mainly, it takes a value, converts the hexadecimal string to binary, reverses the string, and then base64 decodes the string. The last line of the code runs this function, using the same hard-coded $encodedSecret
, which was provided in line 1 of the script. Running the script yields a value of oubWYf2kBq
.
Take the string, and submit it on the main page, to receive the password: Sda6t0vkOPkM8YeOZkAGVhFoaplvlJFd
.
Summary
So we gained some exposure to PHP. We rolled our sleeves up and read through some code, got a better understanding of local file inclusion, and reading server side files. Lastly, we worked with various PHP/OS string manipulation commands.
Alternative Solutions
Natas8-->Natas9
The reversing of the PHP function could also be accomplished using the Linux command line.
[~]$ echo '3d3d516343746d4d6d6c315669563362' | xxd -r -p | rev | base64 -d && echo ""
oubWYf2kBq